Extreme Conditions Modeling - Full Sea State Approach
Extreme conditions modeling consists of identifying the expected extreme (e.g. 100-year) response of some quantity of interest, such as WEC motions or mooring loads. Three different methods of estimating extreme conditions were adapted from WDRT: full sea state approach, contour approach, and MLER design wave. This noteboook presents the full sea state approach.
The full sea state approach consists of the following steps: 1. Take \(N\) samples to represent the sea state. Each sample represents a small area of the sea state and consists of a representative \((H_{s}, T_{e})_i\) pair and a weight \(W_i\) associated with the probability of that sea state area. 2. For each sample \((H_{s}, T_{e})_i\) calculate the short-term (e.g. 3-hours) extreme for the quantity of interest (e.g. WEC motions or mooring tension). 3. Integrate over the entire sea state to obtain the long-term extreme. This is a sum of the products of the weight of each sea state times the short-term extreme.
See more details and equations in
[1] Coe, Ryan G., Carlos A. Michelén Ströfer, Aubrey Eckert-Gallup, and Cédric Sallaberry. 2018. “Full Long-Term Design Response Analysis of a Wave Energy Converter.” Renewable Energy 116: 356–66.
NOTE: Prior to running this example it is recommended to become familiar with environmental_contours_example.ipynb
and short_term_extremes_example.ipynb
since some code blocks are adapted from those examples and used here without the additional description.
We start by importing the relevant modules, including waves.contours
submodule which includes the sampling function, and loads.extreme
which inlcudes the short-term extreme and full sea state integration functions.
[1]:
from mhkit.wave import resource, contours, graphics
from mhkit.loads import extreme
import matplotlib.pyplot as plt
from mhkit.wave.io import ndbc
import pandas as pd
import numpy as np
Obtain and Process NDBC Buouy Data
The first step will be obtaining the environmental data and creating the contours. See environmental_contours_example.ipynb
for more details and explanations of how this is being done in the following code block.
[2]:
parameter = "swden"
buoy_number = "46022"
ndbc_available_data = ndbc.available_data(parameter, buoy_number)
years_of_interest = ndbc_available_data[ndbc_available_data.year < 2013]
filenames = years_of_interest["filename"]
ndbc_requested_data = ndbc.request_data(parameter, filenames)
ndbc_data = {}
for year in ndbc_requested_data:
year_data = ndbc_requested_data[year]
ndbc_data[year] = ndbc.to_datetime_index(parameter, year_data)
Hm0_list = []
Te_list = []
# Iterate over each year and save the result in the initalized dictionary
for year in ndbc_data:
year_data = ndbc_data[year]
Hm0_list.append(resource.significant_wave_height(year_data.T))
Te_list.append(resource.energy_period(year_data.T))
# Concatenate each list of Series into a single Series
Te = pd.concat(Te_list, axis=0)
Hm0 = pd.concat(Hm0_list, axis=0)
# Name each Series and concat into a dataFrame
Te.name = 'Te'
Hm0.name = 'Hm0'
Hm0_Te = pd.concat([Hm0, Te], axis=1)
# Drop any NaNs created from the calculation of Hm0 or Te
Hm0_Te.dropna(inplace=True)
# Sort the DateTime index
Hm0_Te.sort_index(inplace=True)
Hm0_Te_clean = Hm0_Te[Hm0_Te.Hm0 < 20]
Hm0 = Hm0_Te_clean.Hm0.values
Te = Hm0_Te_clean.Te.values
dt = (Hm0_Te_clean.index[2] - Hm0_Te_clean.index[1]).seconds
1. Sampling
The first step is sampling the sea state to get samples \((H_s, T_e)_i\) and associtated weights. For this we will use the waves.contours.samples_full_seastate
function. We will sample 20 points between each return level, for 10 levels ranging from 0.001—100 years return periods. For more details on the sampling approach see
[1] Coe, Ryan G., Carlos A. Michelén Ströfer, Aubrey Eckert-Gallup, and Cédric Sallaberry. 2018. “Full Long-Term Design Response Analysis of a Wave Energy Converter.” Renewable Energy 116: 356–66.
[2] Eckert-Gallup, Aubrey C., Cédric J. Sallaberry, Ann R. Dallman, and Vincent S. Neary. 2016. “Application of Principal Component Analysis (PCA) and Improved Joint Probability Distributions to the Inverse First-Order Reliability Method (I-FORM) for Predicting Extreme Sea States.” Ocean Engineering 112 (January): 307–19.
[3]:
# return levels
levels = np.array([0.001, 0.01, 0.05, 0.1, 0.5, 1, 5, 10, 50, 100])
# points per return level interval
npoints = 20
# Create samples
sample_hs, sample_te, sample_weights = contours.samples_full_seastate(
Hm0, Te, npoints, levels, dt
)
We will now plot the samples alongside the contours. First we will create the different contours using contours.environmental_contours
. See environmental_contours_example.ipynb
for more details on using this function. There are 20 samples, randomly distributed, between each set of return levels.
[4]:
# Create the contours
Te_contours = []
Hm0_contours = []
for period in levels:
copula = contours.environmental_contours(
Hm0, Te, dt, period, "PCA", return_PCA=True
)
Hm0_contours.append(copula["PCA_x1"])
Te_contours.append(copula["PCA_x2"])
# plot
fig, ax = plt.subplots(figsize=(8, 4))
labels = [f"{period}-year Contour" for period in levels]
ax = graphics.plot_environmental_contour(
sample_te,
sample_hs,
Te_contours,
Hm0_contours,
data_label="Samples",
contour_label=labels,
x_label="Energy Period, $Te$ [s]",
y_label="Sig. wave height, $Hm0$ [m]",
ax=ax,
)
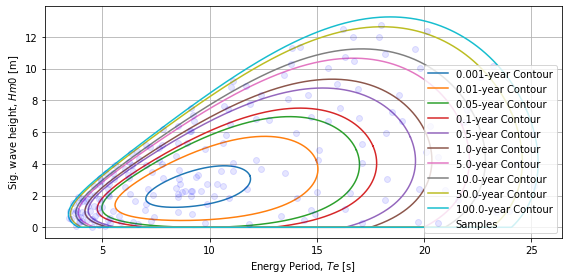
2. Short-Term Extreme Distributions
Many different methods for short-term extremes were adapted from WDRT, and a summary and examples can be found in short_term_extremes_example.ipynb
. The response quantity of interest is typically related to the WEC itself, e.g. maximum heave displacement, PTO extension, or load on the mooring lines. This requires running a simulation (e.g. WEC-Sim) for each of the 200 sampled sea states \((H_s, T_e)_i\). For the sake of example we will consider the wave elevation as the quantity of
interest (can be thought as a proxy for heave motion in this example). Wave elevation time-series for a specific sea state can be created quickly without running any external software.
NOTE: The majority of the for loop below is simply creating the synthetic data (wave elevation time series). In a realistic case the variables time
and data
describing each time series would be obtained externally, e.g. through simulation software such as WEC-Sim or CFD. For this reason the details of creating the synthetic data are not presented here, instead assume for each sea state there is time-series data available.
The last lines of the for-loop create the short-term extreme distribution from the time-series using the loads.extreme.short_term_extreme
function. The short-term period will be 3-hours and we will use 1-hour “simulations” and the Weibul-tail-fitting method to estimate the 3-hour short-term extreme distributions for each of the 200 samples.
For more details on short-term extreme distributions see short_term_extremes_example.ipynb
and
[3] Michelén Ströfer, Carlos A., and Ryan Coe. 2015. “Comparison of Methods for Estimating Short-Term Extreme Response of Wave Energy Converters.” In OCEANS 2015 - MTS/IEEE Washington, 1–6. IEEE.
[5]:
# create the short-term extreme distribution for each sample sea state
t_st = 3.0 * 60.0 * 60.0
gamma = 3.3
t_sim = 1.0 * 60.0 * 60.0
ste_all = []
i = 0
n = len(sample_hs)
for hs, te in zip(sample_hs, sample_te):
tp = te / (0.8255 + 0.03852 * gamma - 0.005537 * gamma**2 + 0.0003154 * gamma**3)
i += 1
print(f"Sea state {i}/{n}. (Hs, Te) = ({hs} m, {te} s). Tp = {tp} s")
# time & frequency arrays
df = 1.0 / t_sim
T_min = tp / 10.0 # s
f_max = 1.0 / T_min
Nf = int(f_max / df) + 1
time = np.linspace(0, t_sim, 2 * Nf + 1)
f = np.linspace(0.0, f_max, Nf)
# spectrum
S = resource.jonswap_spectrum(f, tp, hs, gamma)
# 1-hour elevation time-series
data = resource.surface_elevation(S, time).values.squeeze()
# 3-hour extreme distribution
ste = extreme.short_term_extreme(time, data, t_st, "peaks_weibull_tail_fit")
ste_all.append(ste)
Sea state 1/200. (Hs, Te) = (2.840183371772305 m, 10.181962895642807 s). Tp = 11.267563328978767 s
Sea state 2/200. (Hs, Te) = (2.435150836386068 m, 9.253557835748264 s). Tp = 10.240171762684351 s
Sea state 3/200. (Hs, Te) = (2.380182396284662 m, 9.145665731349082 s). Tp = 10.120776206888838 s
Sea state 4/200. (Hs, Te) = (2.6586225636340433 m, 9.245181682418751 s). Tp = 10.230902544257528 s
Sea state 5/200. (Hs, Te) = (2.489418873168824 m, 9.168527592693843 s). Tp = 10.146075598878438 s
Sea state 6/200. (Hs, Te) = (2.4554983906019254 m, 9.076055379984771 s). Tp = 10.043744002942633 s
Sea state 7/200. (Hs, Te) = (2.8264441787710775 m, 8.352759030940048 s). Tp = 9.243329829171866 s
Sea state 8/200. (Hs, Te) = (2.440636821165454 m, 8.454371440140704 s). Tp = 9.355776148944692 s
Sea state 9/200. (Hs, Te) = (2.2107044601389494 m, 8.139285537198479 s). Tp = 9.00709580097473 s
Sea state 10/200. (Hs, Te) = (1.773654332308055 m, 7.14357692017975 s). Tp = 7.905224775274031 s
Sea state 11/200. (Hs, Te) = (2.33221680310332 m, 9.019225599053808 s). Tp = 9.980855033283783 s
Sea state 12/200. (Hs, Te) = (1.4652901970781362 m, 7.467449653557229 s). Tp = 8.263628805151397 s
Sea state 13/200. (Hs, Te) = (2.37380465141513 m, 9.137972634616318 s). Tp = 10.11226287252288 s
Sea state 14/200. (Hs, Te) = (1.9418617270615008 m, 8.786718919588829 s). Tp = 9.72355850194371 s
Sea state 15/200. (Hs, Te) = (1.682708754224695 m, 9.005540858375955 s). Tp = 9.965711226160469 s
Sea state 16/200. (Hs, Te) = (1.461238938616968 m, 9.679214804474308 s). Tp = 10.711212258578739 s
Sea state 17/200. (Hs, Te) = (1.9389947758035828 m, 10.03218336557303 s). Tp = 11.101814312041364 s
Sea state 18/200. (Hs, Te) = (2.2114494380999004 m, 10.769142843100967 s). Tp = 11.917348376449572 s
Sea state 19/200. (Hs, Te) = (2.3798499882325825 m, 9.899703813443036 s). Tp = 10.955209795926086 s
Sea state 20/200. (Hs, Te) = (2.8107970520086796 m, 10.711433106791901 s). Tp = 11.853485630609148 s
Sea state 21/200. (Hs, Te) = (3.9450344430779625 m, 12.237764463240708 s). Tp = 13.542554368735527 s
Sea state 22/200. (Hs, Te) = (5.208290345944717 m, 12.779909428576214 s). Tp = 14.142502806282762 s
Sea state 23/200. (Hs, Te) = (5.064261814083509 m, 11.637831173755485 s). Tp = 12.878656218475125 s
Sea state 24/200. (Hs, Te) = (4.379521879521117 m, 10.312278693768631 s). Tp = 11.411773391733709 s
Sea state 25/200. (Hs, Te) = (3.5851473474648303 m, 9.202172592956305 s). Tp = 10.183307827579942 s
Sea state 26/200. (Hs, Te) = (3.685482708071981 m, 7.779449820330637 s). Tp = 8.608894415898781 s
Sea state 27/200. (Hs, Te) = (2.9763084662416763 m, 7.623162087670313 s). Tp = 8.435943292098646 s
Sea state 28/200. (Hs, Te) = (2.522737928991046 m, 7.032634458082821 s). Tp = 7.7824536327780285 s
Sea state 29/200. (Hs, Te) = (1.8248226200631286 m, 6.173217780864412 s). Tp = 6.831405987467631 s
Sea state 30/200. (Hs, Te) = (1.5073537401283845 m, 5.798538866794911 s). Tp = 6.416778824161261 s
Sea state 31/200. (Hs, Te) = (1.544648128528352 m, 6.991514600764015 s). Tp = 7.73694957809163 s
Sea state 32/200. (Hs, Te) = (1.1824969929468647 m, 6.59815195035135 s). Tp = 7.301646619300026 s
Sea state 33/200. (Hs, Te) = (1.0552074511072387 m, 7.163058124004591 s). Tp = 7.926783064188546 s
Sea state 34/200. (Hs, Te) = (0.8070976865959585 m, 7.601417023502728 s). Tp = 8.41187977015183 s
Sea state 35/200. (Hs, Te) = (0.43594988466745865 m, 8.895171963861962 s). Tp = 9.843574804997713 s
Sea state 36/200. (Hs, Te) = (1.1925410643512184 m, 9.872043847355096 s). Tp = 10.924600725477926 s
Sea state 37/200. (Hs, Te) = (1.8028237883891736 m, 10.88264042709196 s). Tp = 12.04294706782291 s
Sea state 38/200. (Hs, Te) = (2.0536026727829158 m, 11.313724254083688 s). Tp = 12.519993033371104 s
Sea state 39/200. (Hs, Te) = (2.9552188313626924 m, 13.585806588148326 s). Tp = 15.034324685343892 s
Sea state 40/200. (Hs, Te) = (3.6331136496492835 m, 13.97990050373177 s). Tp = 15.47043687676642 s
Sea state 41/200. (Hs, Te) = (5.144265805980378 m, 16.494051026966694 s). Tp = 18.252646017526228 s
Sea state 42/200. (Hs, Te) = (5.799748193230507 m, 13.531617551407685 s). Tp = 14.97435801590407 s
Sea state 43/200. (Hs, Te) = (5.880641596533005 m, 12.031691730764155 s). Tp = 13.314510170641965 s
Sea state 44/200. (Hs, Te) = (6.51238308153145 m, 12.003103839792574 s). Tp = 13.282874240000137 s
Sea state 45/200. (Hs, Te) = (5.580209865963464 m, 9.727880824002659 s). Tp = 10.765067046955512 s
Sea state 46/200. (Hs, Te) = (4.100873196672923 m, 7.9730435784224145 s). Tp = 8.823129131910912 s
Sea state 47/200. (Hs, Te) = (3.1340604795919393 m, 6.764084114821338 s). Tp = 7.485270463802562 s
Sea state 48/200. (Hs, Te) = (2.56485636049072 m, 5.9144283344779724 s). Tp = 6.545024421760062 s
Sea state 49/200. (Hs, Te) = (1.9133076193222602 m, 5.739115906958378 s). Tp = 6.351020190976688 s
Sea state 50/200. (Hs, Te) = (1.451516130573119 m, 5.529526324054767 s). Tp = 6.1190841760191725 s
Sea state 51/200. (Hs, Te) = (1.1108464969032716 m, 5.620177089281756 s). Tp = 6.219400121822961 s
Sea state 52/200. (Hs, Te) = (0.5408216661607194 m, 5.786678826645898 s). Tp = 6.403654267056419 s
Sea state 53/200. (Hs, Te) = (0.5380468022216697 m, 6.366389882593204 s). Tp = 7.045174090134016 s
Sea state 54/200. (Hs, Te) = (0.3365260257595956 m, 7.308686450335826 s). Tp = 8.087938276228511 s
Sea state 55/200. (Hs, Te) = (0.13904048508259811 m, 9.353861073536656 s). Tp = 10.351169327246874 s
Sea state 56/200. (Hs, Te) = (0.016222817409913045 m, 10.555122094632276 s). Tp = 11.680508745250528 s
Sea state 57/200. (Hs, Te) = (0.9853173366828838 m, 13.099921941116929 s). Tp = 14.496635038748579 s
Sea state 58/200. (Hs, Te) = (1.473244705812314 m, 14.122791226249372 s). Tp = 15.628562601795295 s
Sea state 59/200. (Hs, Te) = (2.75132120738797 m, 16.45603635813758 s). Tp = 18.210578226388876 s
Sea state 60/200. (Hs, Te) = (3.6161379238827065 m, 16.408517026993035 s). Tp = 18.15799238626064 s
Sea state 61/200. (Hs, Te) = (0.06168214841402529 m, 12.829541181563107 s). Tp = 14.197426294576688 s
Sea state 62/200. (Hs, Te) = (0.8144132100052286 m, 15.079313073296817 s). Tp = 16.68706876584456 s
Sea state 63/200. (Hs, Te) = (1.516942605361148 m, 16.537563949182438 s). Tp = 18.30079828558297 s
Sea state 64/200. (Hs, Te) = (3.5189950096049984 m, 17.443166728395703 s). Tp = 19.302956393038976 s
Sea state 65/200. (Hs, Te) = (5.142358500915479 m, 17.095909887023208 s). Tp = 18.918675042607017 s
Sea state 66/200. (Hs, Te) = (6.688164513590967 m, 15.49638894389953 s). Tp = 17.14861324731346 s
Sea state 67/200. (Hs, Te) = (7.38093521608525 m, 13.973338528525257 s). Tp = 15.463175264053787 s
Sea state 68/200. (Hs, Te) = (7.0401547336088806 m, 12.390922649193692 s). Tp = 13.712042273696882 s
Sea state 69/200. (Hs, Te) = (6.78506479000579 m, 11.942048671980722 s). Tp = 13.21530937289815 s
Sea state 70/200. (Hs, Te) = (5.429163429903464 m, 9.382949943110583 s). Tp = 10.38335965080746 s
Sea state 71/200. (Hs, Te) = (4.638215677462449 m, 7.951976580697559 s). Tp = 8.799815971820943 s
Sea state 72/200. (Hs, Te) = (3.844888983563724 m, 7.138788533096206 s). Tp = 7.899925850571548 s
Sea state 73/200. (Hs, Te) = (3.137219896709583 m, 6.146710336025804 s). Tp = 6.802072320033404 s
Sea state 74/200. (Hs, Te) = (2.0531097877647 m, 5.282256705596885 s). Tp = 5.8454506817841 s
Sea state 75/200. (Hs, Te) = (1.400213943382828 m, 4.84359372140781 s). Tp = 5.360017469633588 s
Sea state 76/200. (Hs, Te) = (1.0439873396686965 m, 4.8568106143709855 s). Tp = 5.37464354713959 s
Sea state 77/200. (Hs, Te) = (0.7636698181099354 m, 5.091330002497621 s). Tp = 5.634167382049754 s
Sea state 78/200. (Hs, Te) = (0.5164301455498969 m, 5.271200170082657 s). Tp = 5.833215298942646 s
Sea state 79/200. (Hs, Te) = (0.27764628706602273 m, 5.770502054768426 s). Tp = 6.385752728477266 s
Sea state 80/200. (Hs, Te) = (0.12019246831682381 m, 6.422497059929555 s). Tp = 7.107263412234976 s
Sea state 81/200. (Hs, Te) = (0.9435318150397511 m, 17.686766663680846 s). Tp = 19.57252894264383 s
Sea state 82/200. (Hs, Te) = (1.8095106472743567 m, 18.69492089098288 s). Tp = 20.688172529045467 s
Sea state 83/200. (Hs, Te) = (3.259242038934493 m, 18.12023589435014 s). Tp = 20.052214643504133 s
Sea state 84/200. (Hs, Te) = (4.553386977816094 m, 19.559176338719734 s). Tp = 21.64457485437285 s
Sea state 85/200. (Hs, Te) = (6.079815681228885 m, 17.580622913690455 s). Tp = 19.455068150726802 s
Sea state 86/200. (Hs, Te) = (7.756236359086145 m, 16.334746346569563 s). Tp = 18.0763562791551 s
Sea state 87/200. (Hs, Te) = (8.032314897416262 m, 13.853555311897695 s). Tp = 15.330620766170338 s
Sea state 88/200. (Hs, Te) = (7.437035773606835 m, 12.219920335227009 s). Tp = 13.522807700582689 s
Sea state 89/200. (Hs, Te) = (6.272689262948052 m, 10.341413873554192 s). Tp = 11.444014963098644 s
Sea state 90/200. (Hs, Te) = (5.680501796529699 m, 8.999952214580796 s). Tp = 9.959526721411192 s
Sea state 91/200. (Hs, Te) = (4.295403919706385 m, 7.407648031465147 s). Tp = 8.197451136758348 s
Sea state 92/200. (Hs, Te) = (3.802009467884611 m, 6.735058106015025 s). Tp = 7.45314970322185 s
Sea state 93/200. (Hs, Te) = (2.989442106573119 m, 5.947499898935134 s). Tp = 6.581622074956081 s
Sea state 94/200. (Hs, Te) = (2.1700845237542685 m, 4.95257206736844 s). Tp = 5.48061508201776 s
Sea state 95/200. (Hs, Te) = (1.6133443085967656 m, 4.56969222054859 s). Tp = 5.056912602874126 s
Sea state 96/200. (Hs, Te) = (1.2266847114435944 m, 4.571489478117535 s). Tp = 5.058901483965535 s
Sea state 97/200. (Hs, Te) = (1.1303877451521183 m, 4.660379638074873 s). Tp = 5.157269108843737 s
Sea state 98/200. (Hs, Te) = (0.4872278356806693 m, 4.633757768877119 s). Tp = 5.127808817130256 s
Sea state 99/200. (Hs, Te) = (0.4798540716715929 m, 4.8704655677404585 s). Tp = 5.389754391033024 s
Sea state 100/200. (Hs, Te) = (0.31668721479335277 m, 5.383362621384034 s). Tp = 5.957336505838085 s
Sea state 101/200. (Hs, Te) = (0.9159629695168572 m, 18.536545272385226 s). Tp = 20.51291090900176 s
Sea state 102/200. (Hs, Te) = (2.7901658222821446 m, 19.71513088475056 s). Tp = 21.817157267199796 s
Sea state 103/200. (Hs, Te) = (3.6108764062877143 m, 19.64828596034304 s). Tp = 21.74318533991013 s
Sea state 104/200. (Hs, Te) = (6.753018218293009 m, 19.628479599524695 s). Tp = 21.721267225777854 s
Sea state 105/200. (Hs, Te) = (7.585465267742793 m, 18.93939426620065 s). Tp = 20.958711644709915 s
Sea state 106/200. (Hs, Te) = (8.635760904114186 m, 17.1930692826929 s). Tp = 19.02619356874327 s
Sea state 107/200. (Hs, Te) = (9.05426230998776 m, 15.60503313558586 s). Tp = 17.268841077909393 s
Sea state 108/200. (Hs, Te) = (8.626994106919245 m, 13.664271430098866 s). Tp = 15.121155445270777 s
Sea state 109/200. (Hs, Te) = (8.249276423093754 m, 12.089069676425865 s). Tp = 13.378005750330896 s
Sea state 110/200. (Hs, Te) = (6.546720844163918 m, 9.609606714783245 s). Tp = 10.634182557445284 s
Sea state 111/200. (Hs, Te) = (4.6701612388987925 m, 7.257490338569866 s). Tp = 8.031283637291722 s
Sea state 112/200. (Hs, Te) = (4.247473885467209 m, 6.845251449907407 s). Tp = 7.575091856563489 s
Sea state 113/200. (Hs, Te) = (3.1407263408032344 m, 5.61649903202472 s). Tp = 6.21532991026395 s
Sea state 114/200. (Hs, Te) = (2.551617919566844 m, 5.052936353097902 s). Tp = 5.591680203450128 s
Sea state 115/200. (Hs, Te) = (1.8905541932864423 m, 4.58657204053238 s). Tp = 5.075592148517581 s
Sea state 116/200. (Hs, Te) = (1.5613774797899114 m, 4.417758226719366 s). Tp = 4.88877941334659 s
Sea state 117/200. (Hs, Te) = (0.8528945597991013 m, 4.288719454514684 s). Tp = 4.74598253295999 s
Sea state 118/200. (Hs, Te) = (0.7535671669252131 m, 4.366286078627602 s). Tp = 4.831819307103156 s
Sea state 119/200. (Hs, Te) = (0.3511622723387135 m, 4.5626616143918675 s). Tp = 5.04913239380011 s
Sea state 120/200. (Hs, Te) = (0.23409447683192347 m, 4.831684374307781 s). Tp = 5.346838348472796 s
Sea state 121/200. (Hs, Te) = (0.6567506058522764 m, 20.65466056484252 s). Tp = 22.85685956020476 s
Sea state 122/200. (Hs, Te) = (1.9109048595321103 m, 20.232119606821662 s). Tp = 22.38926730393905 s
Sea state 123/200. (Hs, Te) = (5.150072039646266 m, 21.75628304033487 s). Tp = 24.075936975282378 s
Sea state 124/200. (Hs, Te) = (8.216295742464304 m, 20.537645676815334 s). Tp = 22.72736854999455 s
Sea state 125/200. (Hs, Te) = (8.60693817012891 m, 18.967871991795526 s). Tp = 20.99022566414745 s
Sea state 126/200. (Hs, Te) = (9.398789015485178 m, 16.100608602045437 s). Tp = 17.817254778671455 s
Sea state 127/200. (Hs, Te) = (9.40047294257686 m, 15.347435783673259 s). Tp = 16.98377870773571 s
Sea state 128/200. (Hs, Te) = (8.79993692151442 m, 12.79158147602177 s). Tp = 14.155419326910422 s
Sea state 129/200. (Hs, Te) = (8.177600332494796 m, 11.910657841670044 s). Tp = 13.180571653648933 s
Sea state 130/200. (Hs, Te) = (6.949387711018023 m, 9.748680946742258 s). Tp = 10.78808487786111 s
Sea state 131/200. (Hs, Te) = (5.977891095364696 m, 8.28691724719266 s). Tp = 9.170467997355125 s
Sea state 132/200. (Hs, Te) = (4.51101544283036 m, 6.834717588605634 s). Tp = 7.563434875435892 s
Sea state 133/200. (Hs, Te) = (3.1807875598100446 m, 5.360130005515921 s). Tp = 5.9316268294942605 s
Sea state 134/200. (Hs, Te) = (2.5974274134022024 m, 5.0469024266918066 s). Tp = 5.5850029400776435 s
Sea state 135/200. (Hs, Te) = (1.7390452207300586 m, 4.245268668775884 s). Tp = 4.69789902636861 s
Sea state 136/200. (Hs, Te) = (1.6719550940091157 m, 4.273249097729748 s). Tp = 4.728862727419275 s
Sea state 137/200. (Hs, Te) = (1.2706497306606221 m, 4.121193500467361 s). Tp = 4.560594969105915 s
Sea state 138/200. (Hs, Te) = (0.7236083260991419 m, 3.953915214219135 s). Tp = 4.375481479380716 s
Sea state 139/200. (Hs, Te) = (0.5662113586554597 m, 4.165911158867419 s). Tp = 4.61008042226563 s
Sea state 140/200. (Hs, Te) = (0.22380262429040848 m, 4.428588430704851 s). Tp = 4.900764333201723 s
Sea state 141/200. (Hs, Te) = (0.9422263633698529 m, 21.68156229851667 s). Tp = 23.993249511278247 s
Sea state 142/200. (Hs, Te) = (2.6808185912102096 m, 22.65619752776177 s). Tp = 25.071800305533483 s
Sea state 143/200. (Hs, Te) = (5.284470291643777 m, 22.420384661431555 s). Tp = 24.810845081830916 s
Sea state 144/200. (Hs, Te) = (7.449969927903553 m, 21.84994192365026 s). Tp = 24.179581764592026 s
Sea state 145/200. (Hs, Te) = (9.184267967048088 m, 20.471169146791553 s). Tp = 22.653804295281518 s
Sea state 146/200. (Hs, Te) = (10.717866496341058 m, 17.115719729476954 s). Tp = 18.940597009586504 s
Sea state 147/200. (Hs, Te) = (10.671834020151834 m, 14.880105357839954 s). Tp = 16.46662153258152 s
Sea state 148/200. (Hs, Te) = (10.191452698929544 m, 13.818581919045513 s). Tp = 15.291918511719986 s
Sea state 149/200. (Hs, Te) = (8.37690632915727 m, 10.962421795675256 s). Tp = 12.131234722393874 s
Sea state 150/200. (Hs, Te) = (7.4694526641366625 m, 9.888932685570508 s). Tp = 10.943290250876458 s
Sea state 151/200. (Hs, Te) = (5.212528893273048 m, 7.249094730639045 s). Tp = 8.021992890014861 s
Sea state 152/200. (Hs, Te) = (4.063796625344188 m, 6.135879435017965 s). Tp = 6.790086628839425 s
Sea state 153/200. (Hs, Te) = (3.3787764210161813 m, 5.419027822055873 s). Tp = 5.996804328627211 s
Sea state 154/200. (Hs, Te) = (2.795945700731734 m, 4.958285027601677 s). Tp = 5.486937157818241 s
Sea state 155/200. (Hs, Te) = (1.9272686719719325 m, 4.228829976069561 s). Tp = 4.679707640973425 s
Sea state 156/200. (Hs, Te) = (1.568113495064289 m, 4.032997225028029 s). Tp = 4.462995210682322 s
Sea state 157/200. (Hs, Te) = (1.1822964652570693 m, 3.8685969019191884 s). Tp = 4.281066532399067 s
Sea state 158/200. (Hs, Te) = (0.7856775833759984 m, 3.797389763440326 s). Tp = 4.202267291966823 s
Sea state 159/200. (Hs, Te) = (0.3291798465609936 m, 4.03461070522861 s). Tp = 4.464780720070485 s
Sea state 160/200. (Hs, Te) = (0.15420429246200082 m, 4.3518582866098265 s). Tp = 4.81585322453894 s
Sea state 161/200. (Hs, Te) = (2.0790807412143555 m, 23.553887059988554 s). Tp = 26.065201455959503 s
Sea state 162/200. (Hs, Te) = (2.9005504155626443 m, 23.487521867586448 s). Tp = 25.99176040968044 s
Sea state 163/200. (Hs, Te) = (7.085064893935304 m, 23.617650072612047 s). Tp = 26.135762878167114 s
Sea state 164/200. (Hs, Te) = (10.008361591286254 m, 21.465144157914505 s). Tp = 23.75375687810255 s
Sea state 165/200. (Hs, Te) = (9.97460629881344 m, 20.66827347686875 s). Tp = 22.8719238803088 s
Sea state 166/200. (Hs, Te) = (12.515562162825644 m, 18.442195805046968 s). Tp = 20.408501905631287 s
Sea state 167/200. (Hs, Te) = (11.318704374242229 m, 15.379037771055806 s). Tp = 17.018750097614454 s
Sea state 168/200. (Hs, Te) = (9.831621741618951 m, 12.589522993573661 s). Tp = 13.931817378005688 s
Sea state 169/200. (Hs, Te) = (8.719753268757158 m, 11.001963549202046 s). Tp = 12.174992415931788 s
Sea state 170/200. (Hs, Te) = (6.697280646131268 m, 8.394469020363612 s). Tp = 9.289486935821916 s
Sea state 171/200. (Hs, Te) = (5.9260094336014575 m, 7.621869198633718 s). Tp = 8.434512555290185 s
Sea state 172/200. (Hs, Te) = (4.880617435847239 m, 6.717945332768991 s). Tp = 7.434212366849642 s
Sea state 173/200. (Hs, Te) = (3.876024525693232 m, 5.700254261167271 s). Tp = 6.308015118231136 s
Sea state 174/200. (Hs, Te) = (2.783776276233764 m, 4.665403840101326 s). Tp = 5.162828991067907 s
Sea state 175/200. (Hs, Te) = (2.232050172695443 m, 4.35064714713621 s). Tp = 4.8145129534282445 s
Sea state 176/200. (Hs, Te) = (1.4397061034565646 m, 3.8710858327287654 s). Tp = 4.283820832901415 s
Sea state 177/200. (Hs, Te) = (0.9917868156814885 m, 3.749108919277857 s). Tp = 4.148838746336396 s
Sea state 178/200. (Hs, Te) = (0.8379479685905803 m, 3.6939930215454 s). Tp = 4.08784639402683 s
Sea state 179/200. (Hs, Te) = (0.3649362767346382 m, 3.9015035570249985 s). Tp = 4.317481693615992 s
Sea state 180/200. (Hs, Te) = (0.26122851286335796 m, 3.8182178899126895 s). Tp = 4.2253161123619325 s
Sea state 181/200. (Hs, Te) = (1.85918793399297 m, 24.795749666965783 s). Tp = 27.43947139913466 s
Sea state 182/200. (Hs, Te) = (4.002691094113826 m, 24.82391450267687 s). Tp = 27.470639168382846 s
Sea state 183/200. (Hs, Te) = (8.434042106183172 m, 23.892405089109275 s). Tp = 26.439812262364136 s
Sea state 184/200. (Hs, Te) = (10.54951299048162 m, 22.368492149045327 s). Tp = 24.753419792070545 s
Sea state 185/200. (Hs, Te) = (12.438371651972409 m, 19.928667490533087 s). Tp = 22.0534611364409 s
Sea state 186/200. (Hs, Te) = (12.877658419178392 m, 18.405021605067592 s). Tp = 20.367364194095234 s
Sea state 187/200. (Hs, Te) = (12.20514433636702 m, 15.560744193082908 s). Tp = 17.219830050316762 s
Sea state 188/200. (Hs, Te) = (11.349999320223956 m, 13.573437147754268 s). Tp = 15.020636415762421 s
Sea state 189/200. (Hs, Te) = (8.943249528459614 m, 10.699541456942468 s). Tp = 11.840326093579028 s
Sea state 190/200. (Hs, Te) = (6.974694569119304 m, 8.532352187066413 s). Tp = 9.442071199656622 s
Sea state 191/200. (Hs, Te) = (6.794991647155438 m, 8.321004825044408 s). Tp = 9.208189991248899 s
Sea state 192/200. (Hs, Te) = (4.788840720460295 m, 6.38163862650819 s). Tp = 7.062048654450392 s
Sea state 193/200. (Hs, Te) = (3.2611200777648106 m, 4.933748144257159 s). Tp = 5.459784153057397 s
Sea state 194/200. (Hs, Te) = (2.863068054464451 m, 4.600269207998482 s). Tp = 5.0907497073727574 s
Sea state 195/200. (Hs, Te) = (2.3124781374766226 m, 4.192805730047 s). Tp = 4.639842491434172 s
Sea state 196/200. (Hs, Te) = (1.7972861360694252 m, 3.77507151907285 s). Tp = 4.177569477372569 s
Sea state 197/200. (Hs, Te) = (1.0994765211194881 m, 3.465224057093012 s). Tp = 3.8346860927085795 s
Sea state 198/200. (Hs, Te) = (0.8655202915109887 m, 3.438356041093707 s). Tp = 3.8049534100324585 s
Sea state 199/200. (Hs, Te) = (0.4625658856258066 m, 3.5756134401100295 s). Tp = 3.9568451868576466 s
Sea state 200/200. (Hs, Te) = (0.00764320162167542 m, 3.881423657080205 s). Tp = 4.29526087562771 s
3. Long-Term Extreme Distribution
Finally we integrate the weighted short-term extreme distributions over the entire sea state space to obtain the extreme distribution, assuming a 3-hour sea state coherence. For this we use the loads.extreme.full_seastate_long_term_extreme
function. The integral reduces to a sum over the 200 bins of the weighted short-term extreme distributions.
[6]:
lte = extreme.full_seastate_long_term_extreme(ste_all, sample_weights)
Similar to the short-term extreme functions, the output of long-term extreme function is a probability distribution (scipy.stats.rv_continuous
). This object provides common statistical functions (PDF, CDF, PPF, etc.) and metrics (expected value, median, etc). Here, we will look at the survival function and the 100-year return level.
The value of the survival function at a given return level (e.g. 100-years) may be calculated using the return_year_value
function. This gives a 100-year wave of about 11.1 meters.
[7]:
t_st_hr = t_st / (60.0 * 60.0)
t_return_yr = 100.0
x_t = extreme.return_year_value(lte.ppf, t_return_yr, t_st_hr)
print(f"100-year elevation: {x_t} m")
100-year elevation: 10.542254436353211 m
Finally we plot the survival function and show the 100-year return level (dashed grey lines). The 100-year value is about 11.1 m (where the grey line intersects the x-axis)
[8]:
x = np.linspace(0, 20, 1000)
fig, ax = plt.subplots()
# plot survival function
ax.semilogy(x, lte.sf(x))
# format plot
plt.grid(True, which="major", linestyle=":")
ax.tick_params(axis="both", which="major", direction="in")
ax.xaxis.set_ticks_position("both")
ax.yaxis.set_ticks_position("both")
plt.minorticks_off()
ax.set_xticks([0, 5, 10, 15, 20])
ax.set_yticks(1.0 * 10.0 ** (-1 * np.arange(11)))
ax.set_xlabel("elevation [m]")
ax.set_ylabel("survival function (1-cdf)")
ax.set_xlim([0, x[-1]])
ylim = [1e-10, 1]
ax.set_ylim(ylim)
# 100-year return level
s_t = lte.sf(x_t)
ax.plot([0, x[-1]], [s_t, s_t], "--", color="0.5", linewidth=1)
ax.plot([x_t, x_t], ylim, "--", color="0.5", linewidth=1)
[8]:
[<matplotlib.lines.Line2D at 0x2351c2b0850>]
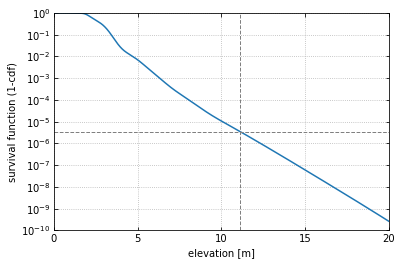